3. Pages & Routes
In the default main.wasp
file created by wasp new
, there is a page and a route declaration:
- JavaScript
- TypeScript
route RootRoute { path: "/", to: MainPage }
page MainPage {
// We specify that the React implementation of the page is exported from
// `src/MainPage.jsx`. This statement uses standard JS import syntax.
// Use `@src` to reference files inside the `src` folder.
component: import { MainPage } from "@src/MainPage"
}
route RootRoute { path: "/", to: MainPage }
page MainPage {
// We specify that the React implementation of the page is exported from
// `src/MainPage.tsx`. This statement uses standard JS import syntax.
// Use `@src` to reference files inside the `src` folder.
component: import { MainPage } from "@src/MainPage"
}
Together, these declarations tell Wasp that when a user navigates to /
, it should render the named export from src/MainPage.tsx
.
The MainPage Component
Let's take a look at the React component referenced by the page declaration:
- JavaScript
- TypeScript
import waspLogo from "./waspLogo.png";
import "./Main.css";
export const MainPage = () => {
// ...
};
import waspLogo from "./waspLogo.png";
import "./Main.css";
export const MainPage = () => {
// ...
};
This is a regular functional React component. It also uses the CSS file and a logo image that sit next to it in the src
folder.
That is all the code you need! Wasp takes care of everything else necessary to define, build, and run the web app.
wasp start
automatically picks up the changes you make, regenerates the code, and restarts the app. So keep it running in the background.
It also improves your experience by tracking the working directory and ensuring the generated code/types are up to date with your changes.
Adding a Second Page
To add more pages, you can create another set of page and route declarations. You can even add parameters to the URL path, using the same syntax as React Router. Let's test this out by adding a new page:
route HelloRoute { path: "/hello/:name", to: HelloPage }
page HelloPage {
component: import { HelloPage } from "@src/HelloPage"
}
When a user visits /hello/their-name
, Wasp renders the component exported from src/HelloPage.tsx
and you can use the useParams
hook from react-router-dom
to access the name
parameter:
- JavaScript
- TypeScript
import { useParams } from "react-router-dom";
export const HelloPage = () => {
const { name } = useParams();
return <div>Here's {name}!</div>;
};
import { useParams } from "react-router-dom";
export const HelloPage = () => {
const { name } = useParams<"name">();
return <div>Here's {name}!</div>;
};
Now you can visit /hello/johnny
and see "Here's johnny!"
Cleaning Up
Now that you've seen how Wasp deals with Routes and Pages, it's finally time to build the Todo app.
Start by cleaning up the starter project and removing unnecessary code and files.
First, remove most of the code from the MainPage
component:
- JavaScript
- TypeScript
export const MainPage = () => {
return <div>Hello world!</div>;
};
export const MainPage = () => {
return <div>Hello world!</div>;
};
At this point, the main page should look like this:
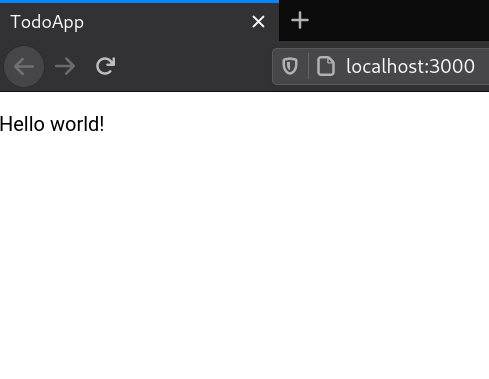
You can now delete redundant files: src/Main.css
, src/waspLogo.png
, and src/HelloPage.tsx
(we won't need this page for the rest of the tutorial).
Since src/HelloPage.tsx
no longer exists, remove its route
and page
declarations from the main.wasp
file.
Your Wasp file should now look like this:
app TodoApp {
wasp: {
version: "^0.17.0"
},
title: "TodoApp"
}
route RootRoute { path: "/", to: MainPage }
page MainPage {
component: import { MainPage } from "@src/MainPage"
}
Excellent work!
You now have a basic understanding of Wasp and are ready to start building your TodoApp. We'll implement the app's core features in the following sections.