Auth UI
To make using authentication in your app as easy as possible, Wasp generates the server-side code but also the client-side UI for you. It enables you to quickly get the login, signup, password reset and email verification flows in your app.
Below we cover all of the available UI components and how to use them.
Overview
After Wasp generates the UI components for your auth, you can use it as is, or customize it to your liking.
Based on the authentication providers you enabled in your main.wasp
file, the Auth UI will show the corresponding UI (form and buttons). For example, if you enabled e-mail authentication:
- JavaScript
- TypeScript
app MyApp {
//...
auth: {
methods: {
email: {},
},
// ...
}
}
app MyApp {
//...
auth: {
methods: {
email: {},
},
// ...
}
}
You'll get the following UI:
And then if you enable Google and Github:
- JavaScript
- TypeScript
app MyApp {
//...
auth: {
methods: {
email: {},
google: {},
github: {},
},
// ...
}
}
app MyApp {
//...
auth: {
methods: {
email: {},
google: {},
github: {},
},
// ...
}
}
The form will automatically update to look like this:
Let's go through all of the available components and how to use them.
Auth Components
The following components are available for you to use in your app:
Login Form
Used with Username & Password, Email, Github and Google authentication.
You can use the LoginForm
component to build your login page:
- JavaScript
- TypeScript
// ...
route LoginRoute { path: "/login", to: LoginPage }
page LoginPage {
component: import { LoginPage } from "@client/LoginPage.jsx"
}
import { LoginForm } from '@wasp/auth/forms/Login'
// Use it like this
export function LoginPage() {
return <LoginForm />
}
// ...
route LoginRoute { path: "/login", to: LoginPage }
page LoginPage {
component: import { LoginPage } from "@client/LoginPage.tsx"
}
import { LoginForm } from '@wasp/auth/forms/Login'
// Use it like this
export function LoginPage() {
return <LoginForm />
}
It will automatically show the correct authentication providers based on your main.wasp
file.
Signup Form
Used with Username & Password, Email, Github and Google authentication.
You can use the SignupForm
component to build your signup page:
- JavaScript
- TypeScript
// ...
route SignupRoute { path: "/signup", to: SignupPage }
page SignupPage {
component: import { SignupPage } from "@client/SignupPage.jsx"
}
import { SignupForm } from '@wasp/auth/forms/Signup'
// Use it like this
export function SignupPage() {
return <SignupForm />
}
// ...
route SignupRoute { path: "/signup", to: SignupPage }
page SignupPage {
component: import { SignupPage } from "@client/SignupPage.tsx"
}
import { SignupForm } from '@wasp/auth/forms/Signup'
// Use it like this
export function SignupPage() {
return <SignupForm />
}
It will automatically show the correct authentication providers based on your main.wasp
file.
Read more about customizing the signup process like adding additional fields or extra UI in the Using Auth section.
Forgot Password Form
Used with Email authentication.
If users forget their password, they can use this form to reset it.
You can use the ForgotPasswordForm
component to build your own forgot password page:
- JavaScript
- TypeScript
// ...
route RequestPasswordResetRoute { path: "/request-password-reset", to: RequestPasswordResetPage }
page RequestPasswordResetPage {
component: import { ForgotPasswordPage } from "@client/ForgotPasswordPage.jsx"
}
import { ForgotPasswordForm } from '@wasp/auth/forms/ForgotPassword'
// Use it like this
export function ForgotPasswordPage() {
return <ForgotPasswordForm />
}
// ...
route RequestPasswordResetRoute { path: "/request-password-reset", to: RequestPasswordResetPage }
page RequestPasswordResetPage {
component: import { ForgotPasswordPage } from "@client/ForgotPasswordPage.tsx"
}
import { ForgotPasswordForm } from '@wasp/auth/forms/ForgotPassword'
// Use it like this
export function ForgotPasswordPage() {
return <ForgotPasswordForm />
}
Reset Password Form
Used with Email authentication.
After users click on the link in the email they receive after submitting the forgot password form, they will be redirected to this form where they can reset their password.
You can use the ResetPasswordForm
component to build your reset password page:
- JavaScript
- TypeScript
// ...
route PasswordResetRoute { path: "/password-reset", to: PasswordResetPage }
page PasswordResetPage {
component: import { ResetPasswordPage } from "@client/ResetPasswordPage.jsx"
}
import { ResetPasswordForm } from '@wasp/auth/forms/ResetPassword'
// Use it like this
export function ResetPasswordPage() {
return <ResetPasswordForm />
}
// ...
route PasswordResetRoute { path: "/password-reset", to: PasswordResetPage }
page PasswordResetPage {
component: import { ResetPasswordPage } from "@client/ResetPasswordPage.tsx"
}
import { ResetPasswordForm } from '@wasp/auth/forms/ResetPassword'
// Use it like this
export function ResetPasswordPage() {
return <ResetPasswordForm />
}
Verify Email Form
Used with Email authentication.
After users sign up, they will receive an email with a link to this form where they can verify their email.
You can use the VerifyEmailForm
component to build your email verification page:
- JavaScript
- TypeScript
// ...
route EmailVerificationRoute { path: "/email-verification", to: EmailVerificationPage }
page EmailVerificationPage {
component: import { VerifyEmailPage } from "@client/VerifyEmailPage.jsx"
}
import { VerifyEmailForm } from '@wasp/auth/forms/VerifyEmail'
// Use it like this
export function VerifyEmailPage() {
return <VerifyEmailForm />
}
// ...
route EmailVerificationRoute { path: "/email-verification", to: EmailVerificationPage }
page EmailVerificationPage {
component: import { VerifyEmailPage } from "@client/VerifyEmailPage.tsx"
}
import { VerifyEmailForm } from '@wasp/auth/forms/VerifyEmail'
// Use it like this
export function VerifyEmailPage() {
return <VerifyEmailForm />
}
Customization 💅🏻
You customize all of the available forms by passing props to them.
Props you can pass to all of the forms:
appearance
- customize the form colors (via design tokens)logo
- path to your logosocialLayout
- layout of the social buttons, which can bevertical
orhorizontal
1. Customizing the Colors
We use Stitches to style the Auth UI. You can customize the styles by overriding the default theme tokens.
See the list of all available tokens which you can override.
- JavaScript
- TypeScript
export const authAppearance = {
colors: {
brand: '#5969b8', // blue
brandAccent: '#de5998', // pink
submitButtonText: 'white',
},
}
import { LoginForm } from '@wasp/auth/forms/Login'
import { authAppearance } from './appearance'
export function LoginPage() {
return (
<LoginForm
// Pass the appearance object to the form
appearance={authAppearance}
/>
)
}
import type { CustomizationOptions } from '@wasp/auth/forms/types'
export const authAppearance: CustomizationOptions['appearance'] = {
colors: {
brand: '#5969b8', // blue
brandAccent: '#de5998', // pink
submitButtonText: 'white',
},
}
import { LoginForm } from '@wasp/auth/forms/Login'
import { authAppearance } from './appearance'
export function LoginPage() {
return (
<LoginForm
// Pass the appearance object to the form
appearance={authAppearance}
/>
)
}
We recommend defining your appearance in a separate file and importing it into your components.
2. Using Your Logo
You can add your logo to the Auth UI by passing the logo
prop to any of the components.
- JavaScript
- TypeScript
import { LoginForm } from '@wasp/auth/forms/Login'
import Logo from './logo.png'
export function LoginPage() {
return (
<LoginForm
// Pass in the path to your logo
logo={Logo}
/>
)
}
import { LoginForm } from '@wasp/auth/forms/Login'
import Logo from './logo.png'
export function LoginPage() {
return (
<LoginForm
// Pass in the path to your logo
logo={Logo}
/>
)
}
3. Social Buttons Layout
You can change the layout of the social buttons by passing the socialLayout
prop to any of the components. It can be either vertical
or horizontal
(default).
If we pass in vertical
:
- JavaScript
- TypeScript
import { LoginForm } from '@wasp/auth/forms/Login'
export function LoginPage() {
return (
<LoginForm
// Pass in the socialLayout prop
socialLayout="vertical"
/>
)
}
import { LoginForm } from '@wasp/auth/forms/Login'
export function LoginPage() {
return (
<LoginForm
// Pass in the socialLayout prop
socialLayout="vertical"
/>
)
}
We get this:
Let's Put Everything Together 🪄
If we provide the logo and custom colors:
- JavaScript
- TypeScript
export const appearance = {
colors: {
brand: '#5969b8', // blue
brandAccent: '#de5998', // pink
submitButtonText: 'white',
},
}
import { LoginForm } from '@wasp/auth/forms/Login'
import { authAppearance } from './appearance'
import todoLogo from './todoLogo.png'
export function LoginPage() {
return <LoginForm appearance={appearance} logo={todoLogo} />
}
import type { CustomizationOptions } from '@wasp/auth/forms/types'
export const appearance: CustomizationOptions['appearance'] = {
colors: {
brand: '#5969b8', // blue
brandAccent: '#de5998', // pink
submitButtonText: 'white',
},
}
import { LoginForm } from '@wasp/auth/forms/Login'
import { authAppearance } from './appearance'
import todoLogo from './todoLogo.png'
export function LoginPage() {
return <LoginForm appearance={appearance} logo={todoLogo} />
}
We get a form looking like this:
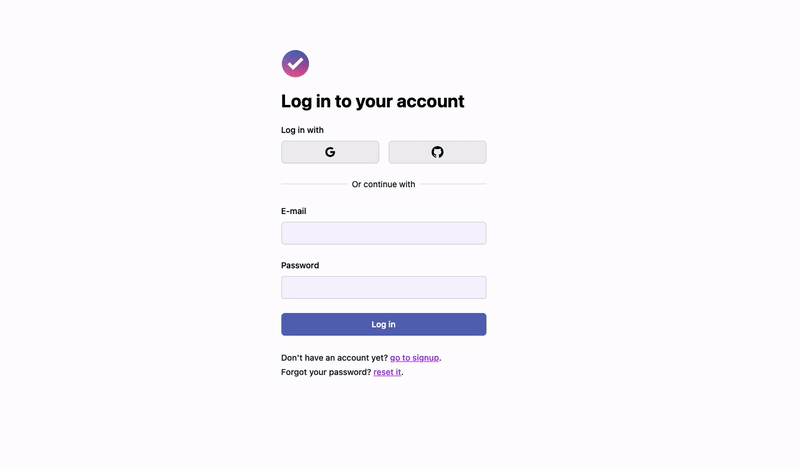